Freefall69
Technical User
I am running Access 2016 and receiving a file from an external system which lists average and max usage for each interface. The challenge is that the information is all listed in one field to include the usage in Kbps, Mbps, and Gbps and need to do a calculation in that field based on value listed and have the result only contain the calculated value in G-bytes. I need to first look at the field and determine if shown in Kbps. Mbps, or Gbps and then based on that perform the calculation and remove test in resulting field. I am uncertain in the best way to accomplish this and would appreciate any assistance to help obtain the desired results. I have attached an image showing the report values received from system and desired output as well. Goal is to convert all values to be in same unit of measurement of Gbps by examining the field and then performing required calculation.
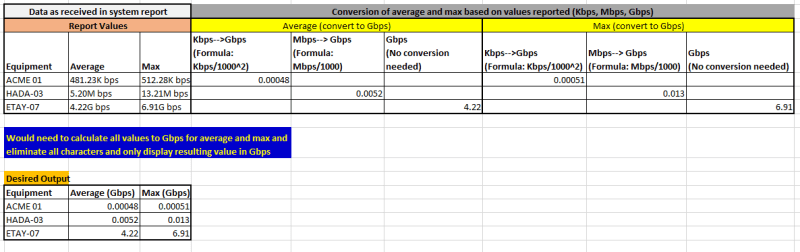