emailx45
Programmer
- Jan 10, 2025
- 44
When working with "exceptions", always keep in mind that it is better to work inside a block away from your code that generates the exception. This way, it is easier to control the flow. You can "eat" it or "export" it to the next level of code.
A basic rule of thumb (mine, of course):
1) use a procedure to "catch" exceptions to handle them in a more user-friendly way
2) if it is not possible to handle it, be explicit in throwing some more user-friendly message.
3) if the "exception" will not generate an "application crash", and, "is not relevant to harm the remaining flow", then, you can "eat" it... however, this is not a good programming practice.
4) do not try to handle an exception inside the exception block itself... this will only confuse the handling system itself. So, do it in a block of code external to the exception code.
Thus, like "pain" in our body, an "exception" can also be seen as something good, as it warns that something is not going well at that point!
The general rule is: always throw an exception to make it clear that something is wrong and should not continue like this!
NOTE: to re-raise your "last exception" on stack use: " RAISE ; "
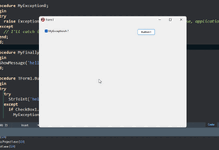
A basic rule of thumb (mine, of course):
1) use a procedure to "catch" exceptions to handle them in a more user-friendly way
2) if it is not possible to handle it, be explicit in throwing some more user-friendly message.
3) if the "exception" will not generate an "application crash", and, "is not relevant to harm the remaining flow", then, you can "eat" it... however, this is not a good programming practice.
4) do not try to handle an exception inside the exception block itself... this will only confuse the handling system itself. So, do it in a block of code external to the exception code.
Thus, like "pain" in our body, an "exception" can also be seen as something good, as it warns that something is not going well at that point!
The general rule is: always throw an exception to make it clear that something is wrong and should not continue like this!
NOTE: to re-raise your "last exception" on stack use: " RAISE ; "
Code:
type
TForm1 = class(TForm)
Button1: TButton;
CheckBox1: TCheckBox;
procedure Button1Click(Sender: TObject);
private
public
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
procedure MyExceptionA;
begin
raise Exception.Create('ERROR A'); // next level... catch me if you can... else, application take it!!!
end;
procedure MyExceptionB;
begin
try
raise Exception.Create('ERROR B'); // next level... catch me if you can... else, application take it!!!
except
// I'll catch it.... so, it's gone! it was eated...
end;
end;
procedure MyFinallyCode;
begin
ShowMessage('hello Finally');
end;
procedure TForm1.Button1Click(Sender: TObject);
begin
try
try
StrToInt('hello');
except
// Preferably identify the correct type of exception if possible. Therefore, always leave the generic type for last.
{
on E:EMathEXCEPTIONS do ...
on E:EConvertEXCEPTIONS do ...
....
on E: EXCEPTION do .... // for last
// YOU CAN use "exception object" to another code...
on E: MyExceptionXXXXX do
myProcExceptions( E );
}
if CheckBox1.Checked then
MyExceptionA
else
MyExceptionB;
end;
finally
MyFinallyCode;
end;
--------
procedure myProcExceptions( XXXX: Exception );
begin
if XXXX = ZZZZ then ....
else
RAISE; // re-raise it
end;
end;
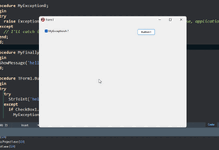
Last edited: