Colleagues,
Subject line says it. Here's the code:
This above procedure is called from this code snippet:
Just in case, AddBackSlash() function (mimics the ADDBS() in VFP)
What's frustrating is that Directory.GetFiles() in the code above does "see" TIF type files, but flat refuses to "see" JPG files - and they are there!
What's even more frustrating is that, when it's run alone, Directory.GetFiles() does see JPGs:
Any idea, anyone, what might cause this behavior?
AHWBGA!
Subject line says it. Here's the code:
Code:
'====================================================================================================================================
Sub GetFilesList(ByRef taFiles() As String, ByVal tsDir As String, tsPattern As String, Optional tlIncludePath As Boolean = False)
'====================================================================================================================================
' Purpose : Fills out the given String Array with the found files' names of the given pattern in the given directory.
' Description : Essentially - wrapper around Directory.GetFiles(Dir as String, File Pattern as String) built-in function.
' The difference is that additional parameter: Include [full] Path flag.
' Parameters : Pointer on the String array to fill out.
' Directory to search as String.
' File name's pattern (e.g. "PO*.XML") to seek.
' Include or not include the full path to the found files, optional, default - just files' names.
' Returns : N/A.
' Side effects : The parameter-array will be resized, all the previous rows will be deleted and replaced with the new ones.
' Notes : 1. Generic.
' 2. Complies with .NET Framework ver. 1.1 and higher, .NET Core ver. 1.0 and higher.
' 3. All String parameters are mandatory.
' 4. String parameters are case-sensitive.
' 5. No String parameters' verification (except if empty), presumed valid.
' Author : Ilya
' Revisions : by Ilya on 2022-06-29 - completed 1st draft.
'====================================================================================================================================
' Check if String params are blank, if so - just return.
If (IsNothing(taFiles) Or IsNothing(tsDir) Or tsDir.Trim() = "" Or IsNothing(tsPattern) Or tsPattern.Trim(tsPattern) = "") Then
Return
End If
' Does directory exist? If not - just return.
If Not Directory.Exists(tsDir) Then Return
' Adjust params
Dim lsDir As String = AddBackSlash(tsDir), lsPattern As String = tsPattern.Trim()
Dim laFiles(0) As String, liCnt As Int32 = 0, lsErrMsg As String = "", llGoOn As Boolean = True
' Let's get 'em!
Try
laFiles = Directory.GetFiles(lsDir, lsPattern)
Catch loErr As Exception
llGoOn = False
lsErrMsg = Read_Exception(loErr)
MsgBox(lsErrMsg, vbCritical, "Error occurred!")
End Try
If Not llGoOn Then Return
' Path or no Path?
If Not tlIncludePath Then
For liCnt = 0 To laFiles.Length() - 1
laFiles(liCnt) = laFiles(liCnt).Replace(lsDir, "")
Next ' liCnt
End If
' And we're done here!
taFiles = laFiles
End Sub
'====================================================================================================================================
This above procedure is called from this code snippet:
Code:
For Each lcExt In gaExtensions
CheckFileSizes(laOversizedImgFiles, giMaxImgSize, gsLastTargetDir, lcExt)
' Were the any oversized image files with this extension?
If laOversizedImgFiles.Length = 0 Then Continue For
' We've gotten oversized files' list for one, current extension only.
' Transfer them to the Totals array, but preserve the current Totals' array's size:
AppendArray1Dim(laTtlOversizedImgFiles, laOversizedImgFiles)
ReDim laOversizedImgFiles(0)
Next
Just in case, AddBackSlash() function (mimics the ADDBS() in VFP)
Code:
'===================================================================================================================================
Public Function AddBackSlash(ByVal tsPath As String) As String
'===================================================================================================================================
' Purpose : Ensures the given path string has backslash at the end.
' Description : Checks if the passed parameter's data type match those of the Function's argument; if not – throws error message
' and returns unchanged parameter.
' Checks if it's an empty string, if it is - returns it As-Is.
' Checks if it's only a file name, if it is - returns it As-Is.
' Checks the given parameter-string in case it's full path to a file, cuts off the file name if it is.
' Checks if the given parameter-string has backslash at the end, adds it if it has not.
' Parameters : Path as String - mandatory
' Returns : Path with the backslash at the end, as String.
' Side effects : None.
' Notes : 1. Generic, applies with .NET Framework ver. 1.1, .NET Core 1.0, .NET Standard 1.0 and higher.
' 2. Verbose on errors, silent otherwise.
' Author : Ilya
' Revisions : 2020-03-09 by Ilya – completed 1st draft.
'===================================================================================================================================
Dim lsPath As String = "", lsRet As String = ""
' Parameter's verification
If VarType(tsPath) <> VariantType.String Then
MsgBox("Invalid parameter passed: " & Chr(34) & "tsPath" & Chr(34) & " must be of type String", MsgBoxStyle.Critical, "Fatal Error: INVALID PARAMETER")
Return lsRet
End If
If String.IsNullOrEmpty(tsPath) Then
Return lsRet
End If
If Path.GetFileName(tsPath) <> "" And Path.GetExtension(tsPath) <> "" Then 'Path + File name? Strip off that latter
lsPath = tsPath.Replace(Path.GetFileName(tsPath), "")
Else
lsPath = tsPath
End If
If String.IsNullOrEmpty(lsPath) Then ' Only the file name was passed? Return blank string
Return lsPath
End If
' Check for the closing backslash
If Strings.Right(lsPath, 1) <> Path.DirectorySeparatorChar Then
lsRet = lsPath & Path.DirectorySeparatorChar
Else
lsRet = lsPath
End If
Return lsRet
End Function
'===================================================================================================================================
What's frustrating is that Directory.GetFiles() in the code above does "see" TIF type files, but flat refuses to "see" JPG files - and they are there!
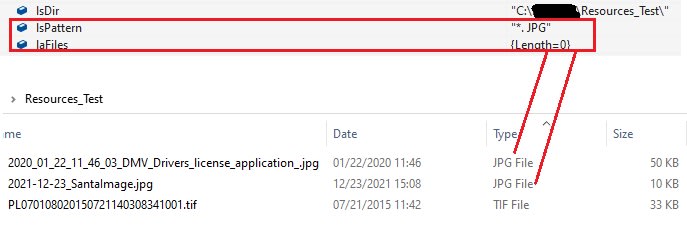
What's even more frustrating is that, when it's run alone, Directory.GetFiles() does see JPGs:
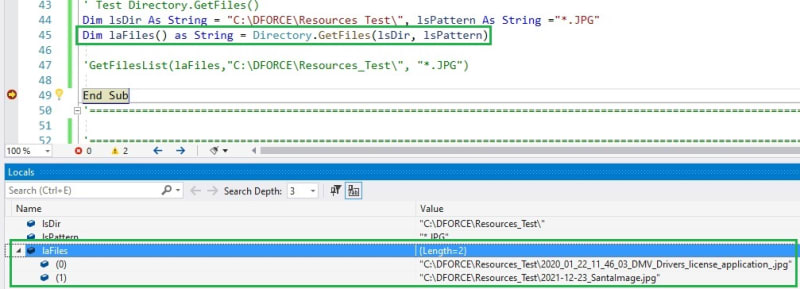
Any idea, anyone, what might cause this behavior?
AHWBGA!