As mentioned in a previous thread post of mine, I am building a custom label which I do my own custom drawing in, rather than the inherited text drawing. It's specifically a 3-D style which I'm doing, but that's besides the point. The fact is I am custom drawing the text into the canvas.
Now my dilemma is figuring out how to mimic these two properties of the label: Alignment and WordWrap.
Supposedly you're able to identify the width of a given text/font before you draw it, which is exactly what I need to be able to do this, but I haven't figured it out yet. I can't simply count the number of characters, because they can vary in size, especially across different fonts. I need to know how wide a given text/font will be before I draw it.
With this ability, I will then need to identify word by word what's the most I can put in one line of text. I should then draw what will fit on the first line, then move on to the next line, and so on until there is no text left to draw.
It's a subject that has me very puzzled, since it requires a lot of match, which I'm horrible at (bad subject to not know well being a programmer).
Basically, I have to try to mimic all the functionality of the inherited label manually.
JD Solutions
Now my dilemma is figuring out how to mimic these two properties of the label: Alignment and WordWrap.
Supposedly you're able to identify the width of a given text/font before you draw it, which is exactly what I need to be able to do this, but I haven't figured it out yet. I can't simply count the number of characters, because they can vary in size, especially across different fonts. I need to know how wide a given text/font will be before I draw it.
With this ability, I will then need to identify word by word what's the most I can put in one line of text. I should then draw what will fit on the first line, then move on to the next line, and so on until there is no text left to draw.
It's a subject that has me very puzzled, since it requires a lot of match, which I'm horrible at (bad subject to not know well being a programmer).
Basically, I have to try to mimic all the functionality of the inherited label manually.
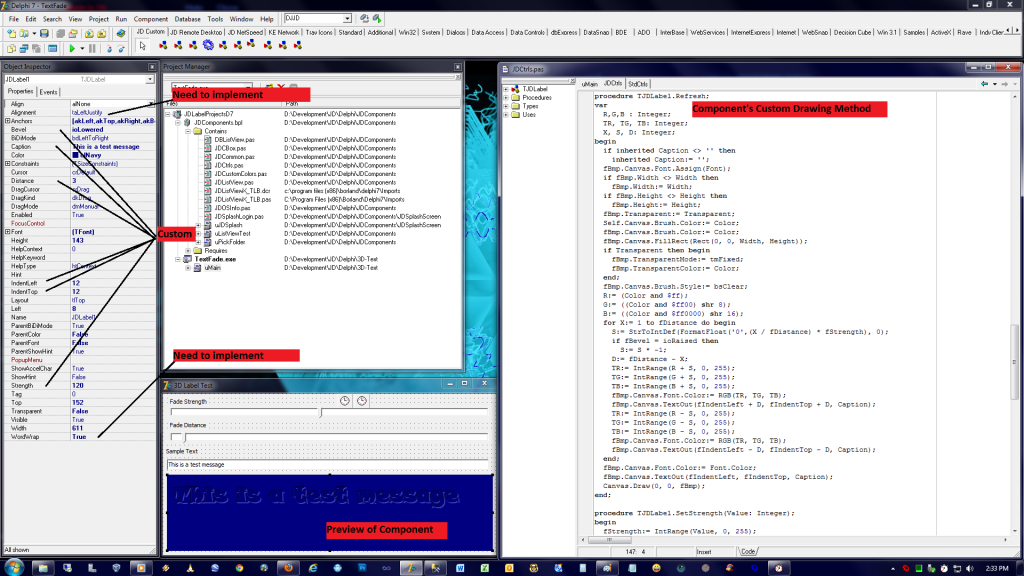
JD Solutions